Day 3! Today, we’ll dive into Python operators and basic input/output operations. We’ll cover six types of operators and learn how to use input and output functions effectively. Even if you’re already familiar with these concepts, revisiting the basics can help solidify your understanding. I might already know most of them, but to keep this challenge authentic and validate my own knowledge, I’ll go through each topic with you step by step.
We will follow the same structure as Day 2. Explain as much as we can about the operators and then share a snippet. These will also be in the Github Repository I created for this challenge. We will also be creating a mini project at the end trying to apply as much of what we learned as possible.
Like I mentioned we will cover six types of operators:
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Logical Operators
- Membership Operators
- Identity Operators
We will also look at “in” and “out” operations
Arithmetic Operators
There are seven basic arithmetic operations: Addition, Subtraction, Multiplication, Division, Modulus, Exponentiation and Floor Division.
The first 4 are the basics that we all know from elementary school. Exponentiation we learn in high school. Floor Division is just about rounding down after a division so nothing new or complex there. Modulus is something I learned when I started studying Computer Science. It basically just return what’s leftover. So if you do 9 % 2
we would have the result of 1
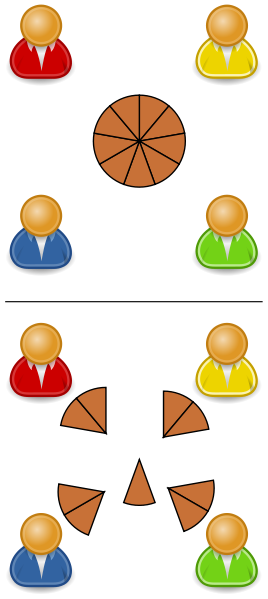
I think this image represents Modulus pretty well.
# Addition
addition = 10 + 5
print("Addition: 10 + 5 =", addition) # Output: 15
# Subtraction
subtraction = 10 - 5
print("Subtraction: 10 - 5 =", subtraction) # Output: 5
# Multiplication
multiplication = 10 * 5
print("Multiplication: 10 * 5 =", multiplication) # Output: 50
# Division
division = 10 / 5
print("Division: 10 / 5 =", division) # Output: 2.0
# Modulus
modulus = 10 % 3
print("Modulus: 10 % 3 =", modulus) # Output: 1
# Exponentiation
exponentiation = 2 ** 3
print("Exponentiation: 2 ** 3 =", exponentiation) # Output: 8
# Floor Division
floor_division = 10 // 3
print("Floor Division: 10 // 3 =", floor_division) # Output: 3
Assignment Operators
The equal sign (=) is the most common assignment operator used to assign a value to a variable. There are a total of thirteen assignment operators. The basic one we just mentioned, the arithmetic ones we saw in the previous section and the Bitwise operators that consist of five new operators (at least for me). We have the Bitwise AND, OR, XOR, Right Shift and Left Shift.
Bitwise operators are a bit more complex to understand. Reading this wiki page I understand they are converted to binary and than the operations are done on a binary level. I recommend reading that wiki page for a more detailed explanation. It helped me a lot to understand it.
# Assignment
a = 15
print("Assignment: a = 15 ->", a)
# Addition Assignment
a += 3
print("Addition Assignment: a += 3 ->", a)
# Subtraction Assignment
a -= 2
print("Subtraction Assignment: a -= 2 ->", a)
# Multiplication Assignment
a *= 4
print("Multiplication Assignment: a *= 4 ->", a)
# Division Assignment
a /= 2
print("Division Assignment: a /= 2 ->", a)
# Modulus Assignment
a %= 5
print("Modulus Assignment: a %= 5 ->", a)
# Floor Division Assignment
a //= 2
print("Floor Division Assignment: a //= 2 ->", a)
# Exponentiation Assignment
a **= 3
print("Exponentiation Assignment: a **= 3 ->", a)
b = 5
# Bitwise AND Assignment
b &= 2
print("Bitwise AND Assignment: b &= 2 ->", b)
b = 5
# Bitwise OR Assignment
b |= 1
print("Bitwise OR Assignment: b |= 1 ->", b)
b = 5
# Bitwise XOR Assignment
b ^= 2
print("Bitwise XOR Assignment: b ^= 2 ->", b)
b = 5
# Bitwise Right Shift Assignment
b >>= 1
print("Bitwise Right Shift Assignment: b >>= 1 ->", b)
b = 5
# Bitwise Left Shift Assignment
b <<= 2
print("Bitwise Left Shift Assignment: b <<= 2 ->", b)
Comparison Operators
Comparison operators in Python are used to compare two values and return a Boolean result—either True or False. The most common comparison operators include == (equals), != (not equals), < (less than), > (greater than), <= (less than or equal to), and >= (greater than or equal to). These operators are essential for controlling the flow of your program, such as making decisions in if statements or loops. Just like assignment operators work at a fundamental level, comparison operators let you evaluate how two values relate to one another, which is crucial when determining the next step in your code.
Much like understanding bitwise operators involves grasping binary-level operations, mastering comparison operators requires you to see how they evaluate expressions and drive logic. When you compare two values, Python checks each operator to decide whether the condition is met, and then returns a Boolean result accordingly. I found that experimenting with these operators in small code snippets helps in grasping their behavior.
# Equal to
a = 5
b = 5
print(a == b) # True
# Not equal to
a = 5
b = 3
print(a != b) # True
# Greater than
a = 7
b = 5
print(a > b) # True
# Less than
a = 3
b = 5
print(a < b) # True
# Greater than or equal to
a = 5
b = 5
print(a >= b) # True
# Less than or equal to
a = 3
b = 5
print(a <= b) # True
# Chaining comparison operators
a = 5
b = 3
c = 7
# Using all different operators in chaining
print(a > b < c) # True, because 5 > 3 and 3 < 7
print(a == b < c) # False, because 5 is not equal to 3
print(a != b >= c) # False, because 3 is not greater than or equal to 7
print(a <= b < c) # False, because 5 is not less than or equal to 3
print(a >= b <= c) # True, because 5 >= 3 and 3 <= 7
Logical Operators
Logical operators in Python are essential for combining multiple conditions to form more complex logical expressions. The three primary logical operators are and, or, and not. The and operator returns True only when both conditions are True, while the or operator returns True if at least one of the conditions is True. The not operator, on the other hand, inverts the Boolean value of a condition, turning True to False and vice versa.
These operators allow you to write concise and readable conditional statements, making your code both efficient and easier to maintain. Experimenting with simple examples can help you understand how logical operators interact and control the flow of your program. As you build more complex decision structures, mastering logical operators will be a key skill in your Python journey.
# and operator
a = True
b = False
print("a and b:", a and b) # False
a = True
b = True
print("a and b:", a and b) # True
# or operator
a = True
b = False
print("a or b:", a or b) # True
a = False
b = False
print("a or b:", a or b) # False
# not operator
a = True
print("not a:", not a) # False
a = False
print("not a:", not a) # True
# Combining logical operators
a = True
b = False
c = True
print("a and b or c:", a and b or c) # True
a = False
b = True
c = False
print("a or b and c:", a or b and c) # False
Membership Operators
Membership operators in Python let you check if a value exists within a collection, like a list, string, or dictionary. Simply put, you can ask Python, “Is this item in that container?” using the in operator, which returns True if the item is found and False if it isn’t. The not in operator does the opposite, telling you when an item isn’t present. This makes your code more intuitive when you’re filtering data or checking for specific entries, without needing lengthy loops or complex logic.
# in operator
print('a' in 'apple') # True
print(1 in [1, 2, 3]) # True
print('key' in {'key': 'value'}) # True
# not in operator
print('b' not in 'apple') # True
print(4 not in [1, 2, 3]) # True
print('another_key' not in {'key': 'value'}) # True
Identity Operators
Identity operators in Python let you check if two variables actually refer to the same object in memory. Instead of comparing values, the is operator verifies if both names point to the exact same item, while is not confirms that they are different objects.
This comes in handy when you need to ensure uniqueness—for example, checking if a variable is None. Using identity operators provides a clear, straightforward way to understand how your data is stored and referenced in your program, reducing the chance for unexpected behavior.
# Using 'is' operator
a = 10
b = 10
print(a is b) # True, because both a and b point to the same memory location
# Using 'is not' operator
c = [1, 2, 3]
d = [1, 2, 3]
print(c is not d) # True, because c and d are different objects in memory
# Using 'is' with None
e = None
print(e is None) # True, because e is None
# 'is not' with None
f = 5
print(f is not None) # True, because f is not None
# Using 'is' with strings
g = "hello"
h = "hello"
print(g is h) # True, because Python optimizes memory usage for small strings
# Using 'is not' with strings
i = "hello"
j = "world"
print(i is not j) # True, because i and j are different strings
Input (In) Operations
The input() function is used to capture data from the user. When your program reaches an input() call, it waits for the user to type something and press Enter. This input is then returned as a string, which you can process or convert as needed.
Output (Out) Operations
The print() function is your go-to for sending information out from your program to the console. It displays messages, variable values, and results, making it easier for you and the user to see what’s happening in your code.
Together, these operations create a basic communication loop in your programs, allowing you to build interactive applications—even simple ones can be engaging with the right input and output!
# Taking input from the user
name = input("Enter your name: ")
age = input("Enter your age: ")
# Printing the input values
print("Your name is:", name)
print("Your age is:", age)
# Performing a simple calculation and displaying the result
num1 = int(input("Enter first number: "))
num2 = int(input("Enter second number: "))
sum = num1 + num2
print("The sum of", num1, "and", num2, "is:", sum)
Exercise
To apply our newly learned operators we will create a simple 2 number calculator. We will have the option of: Addition, Subtraction, Multiplication and Division.
There will be 3 steps:
- Get the user to input two numbers
- User selects the operation
- Perform the operation with the user input and print the result.
Step 1: Obtain two numbers
# Step 1: Get user input for two numbers
num1 = float(input("Enter the first number: "))
num2 = float(input("Enter the second number: "))
We are using float type cast on the input just in case we decide to use decimals. We ask to input each number individually so we can set them in independent variables. This will make everything a lot easier when we get to step 3.
Step 2: Select the operation
# Step 2: User selects the operation
print("Select operation:")
print("1. Addition")
print("2. Subtraction")
print("3. Multiplication")
print("4. Division")
operation = input("Enter the number corresponding to the operation (1/2/3/4): ")
We will be printing the available options so the user knows what the options are. We could probably fit it all in a single print but for readability let’s do it one per line. The input will be defined in a variable so we can us a IF-ELSE
statement to execute the operation in step 3.
Step 3: Execute the operation
# Step 3: Execute the operation
if operation == '1':
result = num1 + num2
print(f"The result of addition is: {result}")
elif operation == '2':
result = num1 - num2
print(f"The result of subtraction is: {result}")
elif operation == '3':
result = num1 * num2
print(f"The result of multiplication is: {result}")
elif operation == '4':
if num2 != 0:
result = num1 / num2
print(f"The result of division is: {result}")
else:
print("Error: Division by zero is not allowed.")
else:
print("Invalid operation selected.")
Comparison Operator is being used to see what the operation variable value is. We are using IF-ELSEIF-ELSE
construct to see the value of the operation variable and act accordingly. In this case we are using the ELSE
clause to notify that the user has selected an invalid operation.
Conclusion
As we saw there are a lot of different operators. We explained all of them and even applied some of them in our exercise. This was a really long post and hopefully you made it to the end. Don’t forgot to check back for Day 4!
Be First to Comment