Welcome back to 30 Days of Python! In Day 3, we learned about operators and basic input/output. Today, we will talk about conditionals, which help programs make decisions.
Conditionals allow a program to run different code depending on certain conditions. This makes programs more dynamic and interactive.
What are conditionals?
Conditionals check if something is True or False. Based on the result, different blocks of code run.
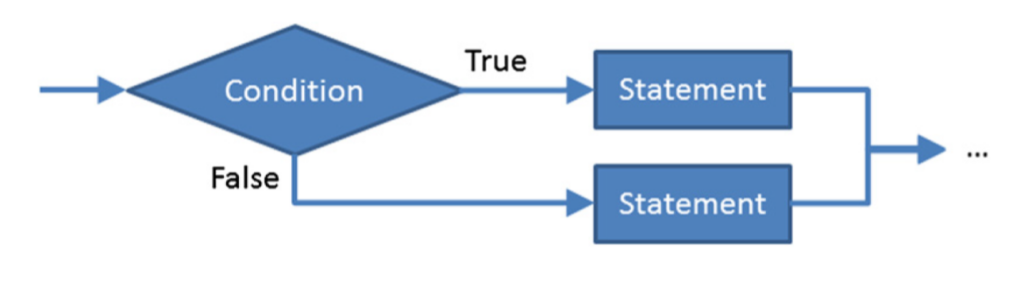
The main conditional statements are:
if
– Runs code only if a condition isTrue
.elif
– Adds more conditions after anif
statement.else
– Runs code if no previous conditions are met.
# if statement
x = 10
if x > 5:
print("x is greater than 5")
# if-else statement
y = 3
if y > 5:
print("y is greater than 5")
else:
print("y is not greater than 5")
# if-elif-else statement
z = 7
if z > 10:
print("z is greater than 10")
elif z > 5:
print("z is greater than 5 but less than or equal to 10")
else:
print("z is 5 or less")
# Nested if statements
a = 15
if a > 10:
if a > 20:
print("a is greater than 20")
else:
print("a is greater than 10 but less than or equal to 20")
else:
print("a is 10 or less")
Using logical operators
We saw logical operators in Day 3. Here’s a quick reminder of how they work:
not
Reverses the conditionand
Both conditions must beTrue
or
At least one condition must beTrue
x = 7
y = 12
# Using 'and' operator
if x > 5 and y > 10:
print("Both x is greater than 5 and y is greater than 10")
else:
print("Either x is not greater than 5 or y is not greater than 10")
# Using 'or' operator
if x > 10 or y > 10:
print("Either x is greater than 10 or y is greater than 10")
else:
print("Neither x is greater than 10 nor y is greater than 10")
# Using 'not' operator
if not x > 10:
print("x is not greater than 10")
else:
print("x is greater than 10")
# Using 'elif' with logical operators
if x > 10 and y > 10:
print("Both x and y are greater than 10")
elif x > 10 and y <= 10:
print("x is greater than 10 and y is 10 or less")
elif x <= 10 and y > 10:
print("x is 10 or less and y is greater than 10")
else:
print("Both x and y are 10 or less")
Ternary Operator
A ternary operation is just a in-line IF-ELSE
statement. In Python they are little different compared to PHP or Javascript.
$Variable = (Condition) ? (Statement1) : (Statement2);
The Above snippet is how PHP and Javascript handle ternary operator. Simple, If Condition
is true, we get Statement1
, if false we get Statement2
.
Variable = "Statement1" if Condition else "Statement2"
As you can see we will still get the same Statements if true or false, but the order of the ternary operation is slightly different. The value we want if the condition is true, comes before the condition and the value if it’s false comes after.
b = 8
result = "b is even" if b % 2 == 0 else "b is odd"
print(result)
Exercise
I’m going to create a simple exercise. I think that Day 3’s exercise is more complex than the one I will do today considering the use of conditional operators.
We will create a little script that will check if we are old enough to vote (18 or older). So the steps to follow are:
- Ask the user for their age.
- Check if they are old enough to vote – 18 or older.
- Print a message based on their age.
Step 1: Ask for the age
age = input("How old are you?")
Ask the user for their age and save it in a variable named age
.
Step 2: Check if they are old enough to vote
if age >= 18:
Make sure they are 18 or older. It’s very important to use >=
to include 18 in our comparison.
Step 3: Print a message based on their age
if age >= 18:
print("You are old enough to vote.")
else:
print("You are a minor.")
As you can see we are using an else statement to print a message if the age introduced is not 18 or over.
Complete snippet:
age = input("How old are you?")
if age >= 18:
print("You are old enough to vote.")
else:
print("You are a minor.")
Conclussions
Day 4 was very simple. I think that conditional statements are pretty easy. We saw a little bit of them in the exercise we did in Day 3 so it’s not that new for ous at this point.
See you in Day 5 where we will look at the different types of loops.
Be First to Comment